It has been already a tradition to cross-post useful information bits, so let me continue.
If you came to the following problem when accessing the the VB Project programmatically from your Perl script:
"Programmatic access to Visual Basic Project is not trusted"
, you will need to allow the access and trust in your target MS Office app:
Office 2003 and Office XP
1. Open the Office 2003 or Office XP application in question. On the Tools menu, click Macro, and then click Security to open the Macro Security dialog box.
2. On the Trusted Sources tab, click to select the Trust access to Visual Basic Project check box to turn on access.
3. Click OK to apply the setting. You may need to restart the application for the code to run properly if you automate from a Component Object Model (COM) add-in or template.
Office 2007
1. Open the 2007 Microsoft Office system application in question. Click the Microsoft Office button, and then click Application Options.
2. Click the Trust Center tab, and then click Trust Center Settings.
3. Click the Macro Settings tab, click to select the Trust access to the VBA project object model check box, and then click OK.
4. Click OK.
Friday, July 31, 2009
Tuesday, July 28, 2009
Java: encoding and listing files in a directory
Two code snippets tested to be working under win32 at least;
Encoding. When your input text files are in encoding different from your default platform's there is no way to use FileReader in this case. Instead you should go deeper in the class hierarchy and specify a file enconding, which you know in advance. Code (inspired by answers here):
Directory listing. Suppose you want to list files in a directory based on some filename criteria. Easy way to do this is to implement a custom filename filter. Entire code (watched here):
Encoding. When your input text files are in encoding different from your default platform's there is no way to use FileReader in this case. Instead you should go deeper in the class hierarchy and specify a file enconding, which you know in advance. Code (inspired by answers here):
private static String loadFileContents(String filename)
throws FileNotFoundException, IOException {
StringBuilder contents = new StringBuilder();
InputStream is =
new BufferedInputStream(new FileInputStream(filename));
Reader reader = new InputStreamReader(is, "UTF8");
BufferedReader bufferedReader =
new BufferedReader(reader);
String line;
while ( (line = bufferedReader.readLine()) != null ) {
contents.append(line + "\n");
}
return contents.toString();
}
Directory listing. Suppose you want to list files in a directory based on some filename criteria. Easy way to do this is to implement a custom filename filter. Entire code (watched here):
/**
*
*/
package org.dk.mt;
import java.io.File;
import java.io.FilenameFilter;
/**
* @author Dmitry_Kan
*
* Lists given directory based on filename pattern
*/
public class DirectoryLister {
private String filenamePattern;
private String dirname;
public DirectoryLister(String filepat, String directory) {
filenamePattern = filepat;
dirname = directory;
}
public String[] getListOfFiles() {
class CustomFilter implements FilenameFilter {
public boolean accept(File dir, String s) {
if (s.contains(filenamePattern) && s.contains("txt") )
return true;
return false;
}
}
return new java.io.File(dirname).list(new CustomFilter());
}
}
Wednesday, July 22, 2009
tabs in Firefox 3.5.1
Just got amazed: it is so easy to drag-n-drop tabs in Firefox 3.5.1. If you have two browser instances opened and you want to drag-n-drop tab from one of them to another, drag the tab and drop it to the tab area of another Firefox instance.
Friday, July 17, 2009
Post builder script for CGI development in Eclipse
Hi,
In CGI development it may be a lot of help to have some sort of a post builder script. The primary aim of this script should be updating the cgi scenario(s) and modules when you build your perl project under Eclipse.
So I have come up with the following post_builder_script.pl:
It is easy to add a builder which will call the script. In Perl perspective go to Project->Properties->Builders. Select "New..." on the right. Choose "Program" in the popup window. Configure the program by choosing what to launch, where and command line arguments:
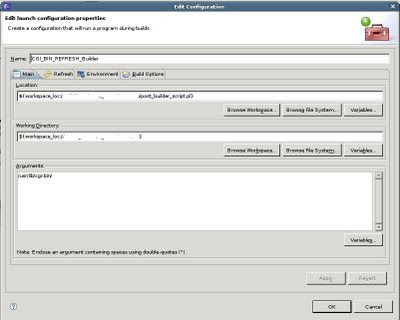
There you go. After the builder has been successfully configured, edit your code and do full rebuild (select the root node of your project and Project->Build Project), when you want to deploy your cgi script set to your web server.
In CGI development it may be a lot of help to have some sort of a post builder script. The primary aim of this script should be updating the cgi scenario(s) and modules when you build your perl project under Eclipse.
So I have come up with the following post_builder_script.pl:
#!/usr/bin/perl -w
use strict;
# append "/" to the target path if required
my $target_path = $ARGV[0];
if (not( $target_path =~ m/\/$/)) {
$target_path .= "/";
}
# copy cgi script: what, from where, to where
my $file = "uni_search.cgi";
my $copy_base_path = "../";
my $copy_from = $copy_base_path.$file;
my $copy_to = $target_path.$file;
copy($copy_from, $copy_to);
# copy Perl modules
my @modules = ("Module1.pm", "Module2.pm", "Module3.pm");
my $modules_path = "some_path/";
$copy_base_path = "./";
my $target_copy_base_path = $target_path.$modules_path;
foreach my $module(@modules) {
copy($copy_base_path.$module,
$target_copy_base_path.$module);
}
sub copy
{
my $copy_from = shift;
my $copy_to = shift;
my $result;
# chmod'ing
$result = system("echo ".[your_sudo_password_here].
" | sudo -S chmod +w ".$copy_to);
print "Changing write rights: ".$result."\n";
# updates / copies the cgi script to server
$result = system("sudo cp ".$copy_from." ".$copy_to);
if ($result == 0) {
print "Result of copying the ".$copy_from.
" to server's cgi-bin: ".$result."\n\n";
}
}
It is easy to add a builder which will call the script. In Perl perspective go to Project->Properties->Builders. Select "New..." on the right. Choose "Program" in the popup window. Configure the program by choosing what to launch, where and command line arguments:
There you go. After the builder has been successfully configured, edit your code and do full rebuild (select the root node of your project and Project->Build Project), when you want to deploy your cgi script set to your web server.
Thursday, July 9, 2009
Information retrieval: conceptual problem
From my experience with grep for example, I come to a conclusion that the main problem of current search engine
interfaces (not grep ones as grep serves other aims) is that they lack one major feature. It is the feature of visualizing the search space or from other perspective -- a feature of search hints.
Suppose I want to search *something* about HTTP protocol (some very specific detail) and I know very little about HTTP (an artificial example). So for example, I even don't know which category does that particular detail belongs too.
Needless to say, that I don't know the term which I'm looking for. Now I'm stuck. I should have some starting hints, which would lead me to first query terms apart from HTTP. Because HTTP is very wide term in a tree of terms (like the tree root), I will spend hours and hours reading through millions of returned hits.
Instead, I would use a concept graph, visualized, which directly corresponds to the search index of a searching engine.
Now I can easily jump in a concise way over those categories getting deeper and deeper into HTTP topic and discovering
some previously unknown terms, which I can further search for. Thus narrowing down the search space with the parallel learning I would come to a result faster.
interfaces (not grep ones as grep serves other aims) is that they lack one major feature. It is the feature of visualizing the search space or from other perspective -- a feature of search hints.
Suppose I want to search *something* about HTTP protocol (some very specific detail) and I know very little about HTTP (an artificial example). So for example, I even don't know which category does that particular detail belongs too.
Needless to say, that I don't know the term which I'm looking for. Now I'm stuck. I should have some starting hints, which would lead me to first query terms apart from HTTP. Because HTTP is very wide term in a tree of terms (like the tree root), I will spend hours and hours reading through millions of returned hits.
Instead, I would use a concept graph, visualized, which directly corresponds to the search index of a searching engine.
Now I can easily jump in a concise way over those categories getting deeper and deeper into HTTP topic and discovering
some previously unknown terms, which I can further search for. Thus narrowing down the search space with the parallel learning I would come to a result faster.
Subscribe to:
Posts (Atom)